Chaining completable futures based on conditionsEclipse/Java code completion not workingBeginner's Java - Help Needed Using EclipseFIlling a 2D array with random integers javaJava HiLo program stopssum of integers. add them. check if sum is odd or even. print the sum of digits and also print if odd or evenjava completable futures and exception chainingRandom Number Sort ArraysMigrating async task executor to use chained CompletableFutureFell on the goallineHow to handle error responses from in any step of a completable future chain?
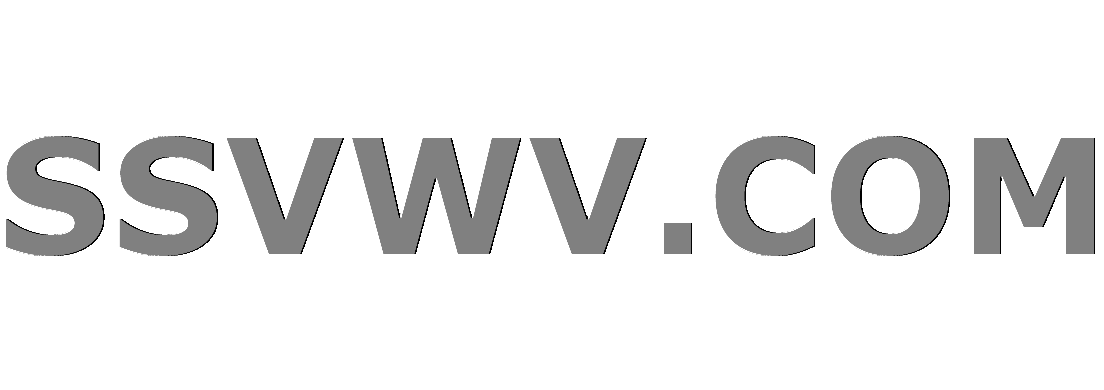
Multi tool use
Can an x86 CPU running in real mode be considered to be basically an 8086 CPU?
What do the dots in this tr command do: tr .............A-Z A-ZA-Z <<< "JVPQBOV" (with 13 dots)
Email Account under attack (really) - anything I can do?
How does strength of boric acid solution increase in presence of salicylic acid?
What do you call a Matrix-like slowdown and camera movement effect?
The use of multiple foreign keys on same column in SQL Server
Is this a crack on the carbon frame?
Is it possible to do 50 km distance without any previous training?
Have astronauts in space suits ever taken selfies? If so, how?
If I cast Expeditious Retreat, can I Dash as a bonus action on the same turn?
Why not use SQL instead of GraphQL?
What's the point of deactivating Num Lock on login screens?
How can I make my BBEG immortal short of making them a Lich or Vampire?
I’m planning on buying a laser printer but concerned about the life cycle of toner in the machine
Is a tag line useful on a cover?
Can a Warlock become Neutral Good?
Writing rule stating superpower from different root cause is bad writing
Why are electrically insulating heatsinks so rare? Is it just cost?
Maximum likelihood parameters deviate from posterior distributions
Dragon forelimb placement
How much RAM could one put in a typical 80386 setup?
Why dont electromagnetic waves interact with each other?
Fencing style for blades that can attack from a distance
Why do falling prices hurt debtors?
Chaining completable futures based on conditions
Eclipse/Java code completion not workingBeginner's Java - Help Needed Using EclipseFIlling a 2D array with random integers javaJava HiLo program stopssum of integers. add them. check if sum is odd or even. print the sum of digits and also print if odd or evenjava completable futures and exception chainingRandom Number Sort ArraysMigrating async task executor to use chained CompletableFutureFell on the goallineHow to handle error responses from in any step of a completable future chain?
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I am having a bunch of methods that return a CompletableFuture and I would like to chain in a specific way
package com.sandbox;
import java.util.Random;
import java.util.concurrent.CompletableFuture;
import java.util.stream.IntStream;
public class SandboxFutures
public CompletableFuture<Integer> generateRandom(int min, int max)
return CompletableFuture.supplyAsync(() ->
if (min >= max)
throw new IllegalArgumentException("max must be greater than min");
Random r = new Random();
return r.nextInt((max - min) + 1) + min;
);
public CompletableFuture<String> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
if (result % 2 == 0)
return "Even";
else
return "Odd";
);
public CompletableFuture<Integer> findFactorial(int evenNumber)
return CompletableFuture.supplyAsync(() ->
if (evenNumber <= 0)
return 0;
return IntStream.rangeClosed(2, evenNumber).reduce(1, (x,y) -> x*y);
);
public CompletableFuture<Integer> convertToNearestEvenInteger(int oddNumber)
return CompletableFuture.supplyAsync(() ->
if (oddNumber <= 0)
return 2;
return oddNumber+1;
);
I am trying to combine them based on the following rules,
- Generate a random number between 1 and 100
- If the number is even print
Even
, if it is odd printOdd
- If the number is even call the
findFactorial
with the random number - If the number is odd find the nearest even via
convertToNearestEvenInteger
I am not too clear on how to do the conditional chaining and exception handling. Some examples or code snippets may be helpful.
java asynchronous exception completable-future conditional-execution
add a comment |
I am having a bunch of methods that return a CompletableFuture and I would like to chain in a specific way
package com.sandbox;
import java.util.Random;
import java.util.concurrent.CompletableFuture;
import java.util.stream.IntStream;
public class SandboxFutures
public CompletableFuture<Integer> generateRandom(int min, int max)
return CompletableFuture.supplyAsync(() ->
if (min >= max)
throw new IllegalArgumentException("max must be greater than min");
Random r = new Random();
return r.nextInt((max - min) + 1) + min;
);
public CompletableFuture<String> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
if (result % 2 == 0)
return "Even";
else
return "Odd";
);
public CompletableFuture<Integer> findFactorial(int evenNumber)
return CompletableFuture.supplyAsync(() ->
if (evenNumber <= 0)
return 0;
return IntStream.rangeClosed(2, evenNumber).reduce(1, (x,y) -> x*y);
);
public CompletableFuture<Integer> convertToNearestEvenInteger(int oddNumber)
return CompletableFuture.supplyAsync(() ->
if (oddNumber <= 0)
return 2;
return oddNumber+1;
);
I am trying to combine them based on the following rules,
- Generate a random number between 1 and 100
- If the number is even print
Even
, if it is odd printOdd
- If the number is even call the
findFactorial
with the random number - If the number is odd find the nearest even via
convertToNearestEvenInteger
I am not too clear on how to do the conditional chaining and exception handling. Some examples or code snippets may be helpful.
java asynchronous exception completable-future conditional-execution
add a comment |
I am having a bunch of methods that return a CompletableFuture and I would like to chain in a specific way
package com.sandbox;
import java.util.Random;
import java.util.concurrent.CompletableFuture;
import java.util.stream.IntStream;
public class SandboxFutures
public CompletableFuture<Integer> generateRandom(int min, int max)
return CompletableFuture.supplyAsync(() ->
if (min >= max)
throw new IllegalArgumentException("max must be greater than min");
Random r = new Random();
return r.nextInt((max - min) + 1) + min;
);
public CompletableFuture<String> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
if (result % 2 == 0)
return "Even";
else
return "Odd";
);
public CompletableFuture<Integer> findFactorial(int evenNumber)
return CompletableFuture.supplyAsync(() ->
if (evenNumber <= 0)
return 0;
return IntStream.rangeClosed(2, evenNumber).reduce(1, (x,y) -> x*y);
);
public CompletableFuture<Integer> convertToNearestEvenInteger(int oddNumber)
return CompletableFuture.supplyAsync(() ->
if (oddNumber <= 0)
return 2;
return oddNumber+1;
);
I am trying to combine them based on the following rules,
- Generate a random number between 1 and 100
- If the number is even print
Even
, if it is odd printOdd
- If the number is even call the
findFactorial
with the random number - If the number is odd find the nearest even via
convertToNearestEvenInteger
I am not too clear on how to do the conditional chaining and exception handling. Some examples or code snippets may be helpful.
java asynchronous exception completable-future conditional-execution
I am having a bunch of methods that return a CompletableFuture and I would like to chain in a specific way
package com.sandbox;
import java.util.Random;
import java.util.concurrent.CompletableFuture;
import java.util.stream.IntStream;
public class SandboxFutures
public CompletableFuture<Integer> generateRandom(int min, int max)
return CompletableFuture.supplyAsync(() ->
if (min >= max)
throw new IllegalArgumentException("max must be greater than min");
Random r = new Random();
return r.nextInt((max - min) + 1) + min;
);
public CompletableFuture<String> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
if (result % 2 == 0)
return "Even";
else
return "Odd";
);
public CompletableFuture<Integer> findFactorial(int evenNumber)
return CompletableFuture.supplyAsync(() ->
if (evenNumber <= 0)
return 0;
return IntStream.rangeClosed(2, evenNumber).reduce(1, (x,y) -> x*y);
);
public CompletableFuture<Integer> convertToNearestEvenInteger(int oddNumber)
return CompletableFuture.supplyAsync(() ->
if (oddNumber <= 0)
return 2;
return oddNumber+1;
);
I am trying to combine them based on the following rules,
- Generate a random number between 1 and 100
- If the number is even print
Even
, if it is odd printOdd
- If the number is even call the
findFactorial
with the random number - If the number is odd find the nearest even via
convertToNearestEvenInteger
I am not too clear on how to do the conditional chaining and exception handling. Some examples or code snippets may be helpful.
java asynchronous exception completable-future conditional-execution
java asynchronous exception completable-future conditional-execution
edited Mar 8 at 4:48
John Kugelman
248k54406460
248k54406460
asked Mar 8 at 4:24
g0c00l.g33kg0c00l.g33k
71811132
71811132
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The way printEvenOrOdd
is written makes it more difficult than it needs to be. The problem is that it doesn't print the word "Even" or "Odd", it returns it, which means the original result
is lost. The rest of the steps rely on having the actual number. To work around it, you could use call printEvenOrOdd
and use .thenApply(__ -> result)
to restore the original number afterwards. It would look like this:
System.out.println(
generateRandom(1, 100)
.thenCompose(result ->
printEvenOrOdd(result)
.thenAccept(System.out::println)
.thenApply(__ -> result)
)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
A better solution would be to change the definition of printEvenOrOdd
to something like:
public CompletableFuture<Integer> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
System.out.println(result % 2 == 0 ? "Even" : "Odd");
return result;
);
That would make it much easier to chain steps 3 and 4:
System.out.println(
generateRandom(1, 100)
.thenApply(this::printEvenOrOdd)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
add a comment |
You can use thenCompose()
:
CompletableFuture<Integer> n = generateRandom(1, 100)
.thenCompose(i -> printEvenOrOdd(i)
.thenCompose(s -> s.equals("Even")
? findFactorial(i)
: convertToNearestEvenInteger(i)));
System.out.println(n.get());
However, when big even numbers are generated, your factorial method can't store anything bigger than int
, so you need to update that.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55056706%2fchaining-completable-futures-based-on-conditions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The way printEvenOrOdd
is written makes it more difficult than it needs to be. The problem is that it doesn't print the word "Even" or "Odd", it returns it, which means the original result
is lost. The rest of the steps rely on having the actual number. To work around it, you could use call printEvenOrOdd
and use .thenApply(__ -> result)
to restore the original number afterwards. It would look like this:
System.out.println(
generateRandom(1, 100)
.thenCompose(result ->
printEvenOrOdd(result)
.thenAccept(System.out::println)
.thenApply(__ -> result)
)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
A better solution would be to change the definition of printEvenOrOdd
to something like:
public CompletableFuture<Integer> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
System.out.println(result % 2 == 0 ? "Even" : "Odd");
return result;
);
That would make it much easier to chain steps 3 and 4:
System.out.println(
generateRandom(1, 100)
.thenApply(this::printEvenOrOdd)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
add a comment |
The way printEvenOrOdd
is written makes it more difficult than it needs to be. The problem is that it doesn't print the word "Even" or "Odd", it returns it, which means the original result
is lost. The rest of the steps rely on having the actual number. To work around it, you could use call printEvenOrOdd
and use .thenApply(__ -> result)
to restore the original number afterwards. It would look like this:
System.out.println(
generateRandom(1, 100)
.thenCompose(result ->
printEvenOrOdd(result)
.thenAccept(System.out::println)
.thenApply(__ -> result)
)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
A better solution would be to change the definition of printEvenOrOdd
to something like:
public CompletableFuture<Integer> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
System.out.println(result % 2 == 0 ? "Even" : "Odd");
return result;
);
That would make it much easier to chain steps 3 and 4:
System.out.println(
generateRandom(1, 100)
.thenApply(this::printEvenOrOdd)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
add a comment |
The way printEvenOrOdd
is written makes it more difficult than it needs to be. The problem is that it doesn't print the word "Even" or "Odd", it returns it, which means the original result
is lost. The rest of the steps rely on having the actual number. To work around it, you could use call printEvenOrOdd
and use .thenApply(__ -> result)
to restore the original number afterwards. It would look like this:
System.out.println(
generateRandom(1, 100)
.thenCompose(result ->
printEvenOrOdd(result)
.thenAccept(System.out::println)
.thenApply(__ -> result)
)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
A better solution would be to change the definition of printEvenOrOdd
to something like:
public CompletableFuture<Integer> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
System.out.println(result % 2 == 0 ? "Even" : "Odd");
return result;
);
That would make it much easier to chain steps 3 and 4:
System.out.println(
generateRandom(1, 100)
.thenApply(this::printEvenOrOdd)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
The way printEvenOrOdd
is written makes it more difficult than it needs to be. The problem is that it doesn't print the word "Even" or "Odd", it returns it, which means the original result
is lost. The rest of the steps rely on having the actual number. To work around it, you could use call printEvenOrOdd
and use .thenApply(__ -> result)
to restore the original number afterwards. It would look like this:
System.out.println(
generateRandom(1, 100)
.thenCompose(result ->
printEvenOrOdd(result)
.thenAccept(System.out::println)
.thenApply(__ -> result)
)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
A better solution would be to change the definition of printEvenOrOdd
to something like:
public CompletableFuture<Integer> printEvenOrOdd(int result)
return CompletableFuture.supplyAsync(() ->
System.out.println(result % 2 == 0 ? "Even" : "Odd");
return result;
);
That would make it much easier to chain steps 3 and 4:
System.out.println(
generateRandom(1, 100)
.thenApply(this::printEvenOrOdd)
.thenCompose(result ->
result % 2 == 0
? findFactorial(result)
: convertToNearestEvenInteger(result)
)
.join()
);
edited Mar 9 at 3:23
answered Mar 8 at 5:35
John KugelmanJohn Kugelman
248k54406460
248k54406460
add a comment |
add a comment |
You can use thenCompose()
:
CompletableFuture<Integer> n = generateRandom(1, 100)
.thenCompose(i -> printEvenOrOdd(i)
.thenCompose(s -> s.equals("Even")
? findFactorial(i)
: convertToNearestEvenInteger(i)));
System.out.println(n.get());
However, when big even numbers are generated, your factorial method can't store anything bigger than int
, so you need to update that.
add a comment |
You can use thenCompose()
:
CompletableFuture<Integer> n = generateRandom(1, 100)
.thenCompose(i -> printEvenOrOdd(i)
.thenCompose(s -> s.equals("Even")
? findFactorial(i)
: convertToNearestEvenInteger(i)));
System.out.println(n.get());
However, when big even numbers are generated, your factorial method can't store anything bigger than int
, so you need to update that.
add a comment |
You can use thenCompose()
:
CompletableFuture<Integer> n = generateRandom(1, 100)
.thenCompose(i -> printEvenOrOdd(i)
.thenCompose(s -> s.equals("Even")
? findFactorial(i)
: convertToNearestEvenInteger(i)));
System.out.println(n.get());
However, when big even numbers are generated, your factorial method can't store anything bigger than int
, so you need to update that.
You can use thenCompose()
:
CompletableFuture<Integer> n = generateRandom(1, 100)
.thenCompose(i -> printEvenOrOdd(i)
.thenCompose(s -> s.equals("Even")
? findFactorial(i)
: convertToNearestEvenInteger(i)));
System.out.println(n.get());
However, when big even numbers are generated, your factorial method can't store anything bigger than int
, so you need to update that.
edited Mar 8 at 5:04
answered Mar 8 at 4:34


KartikKartik
4,52231537
4,52231537
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55056706%2fchaining-completable-futures-based-on-conditions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ke8nL q3qVb g opCvT1krb2nGy4oqcfgAvlTtIRhN5S1DMtJni8992r,l14azQCE,T,bE824mon